やりたいこと
下の記事でNodeJSでGeminiを使用できるようにしました。
しかしGUIがなく実用性は皆無なのでGUIを実装します。
おさらい
前回作成したHTMLは以下の通りです。
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Gemini</title>
</head>
<body>
<script type="module" src="main.js"></script>
</body>
</html>
同様にJSは以下の通りです。
let api_key = "Your API key"
import { GoogleGenerativeAI } from "@google/generative-ai";
const genAI = new GoogleGenerativeAI(api_key);
const model = genAI.getGenerativeModel({ model: "gemini-1.5-flash" });
const prompt = "GoogleのAIのGeminiに関して教えて。";
const result = await model.generateContent(prompt);
console.log(result.response.text());
実装
inputの実装
HTMLの<body>タグに以下を追加してテキストを入力できるようにします。
<div>
<label>INPUT: </label>
<input type="text" id="input" size="100" />
</div>
<div>
<button id="run">実行</button>
</div>

JavaScriptでは上で作成した”実行”ボタンをクリックされたときにプロンプトがAIに渡されるようにします。
import { GoogleGenerativeAI } from "@google/generative-ai";
//初期化
const genAI = new GoogleGenerativeAI(api_key);
const model = genAI.getGenerativeModel({ model: "gemini-1.5-flash" });
//ボタンクリック時に返事の作成
async function generateReply(){
const prompt = document.getElementById("input").value;
const result = await model.generateContent(prompt);
console.log(result.response.text());
}
//ボタンクリック時の動作の指定
document.getElementById("run").onclick = () => generateReply();
outputの実装
HTMLの<body>タグに以下を追加して出力を表示する領域を作成します。
<div id = output>
</div>
JavaScriptでは上で作成したリプライを上記の領域に表示します。
//console.log(result.response.text()); //削除
document.getElementById("output").innerHTML = (result.response.text()); //追加
Markdown対応
ここまでの実装で最低限は動作するのですが、下のようにアウトプットが整形されません。
これは出力がMarkdownという形式で出力されるためです。

ここではunifiedというライブラリを使用してHTMLに変換して表示します。
GitHub - unifiedjs/unified: ☔️ interface for parsing, inspecting, transforming, and serializing content through syntax trees
☔️ interface for parsing, inspecting, transforming, and serializing content through syntax trees - unifiedjs/unified
unifiedのインストール
以下のコマンドを実行してunifiedと使用するモジュールをインストールします。
npm install unified
npm install remark-parse
npm install remark-rehype
npm install rehype-stringify
MarkdownからHTMLへ変換
必要なモジュールをインポートします。
import { unified } from 'unified';
import remarkParse from 'remark-parse'
import remark2rehype from 'remark-rehype'
import rehypeStringify from 'rehype-stringify';
以下のようにMarkdownで書かれたresult.response.text()、をprocessor.processSyncでHTMLに変換しています。
const result = await model.generateContent(prompt);
//ここから追加
const processor = unified().use(remarkParse).use(remark2rehype).use(rehypeStringify); //処理の定義 markdownのパース → HTMLに変換 → ビルド
const html = processor.processSync(result.response.text());//変換
//ここまで追加
//document.getElementById("output").innerHTML = (result.response.text()); //削除
document.getElementById("output").innerHTML = (html.value);//追加
結果
テキストを入力してGeminiから出力を得て表示するようにできました。
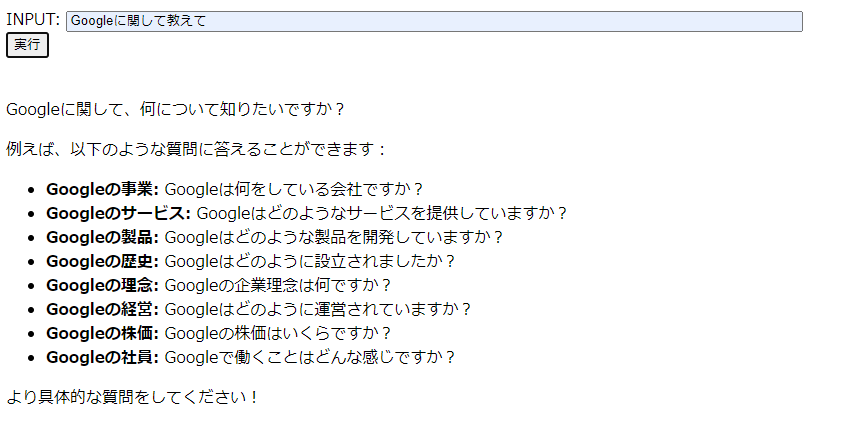
全コード
index.tml
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Gemini</title>
</head>
<body>
<div>
<label>INPUT: </label>
<input type="text" id="input" size="100" />
</div>
<div>
<button id="run">実行</button>
</div>
<script type="module" src="main.js"></script>
<br>
<div id = output>
</div>
</body>
</html>
main.js
let api_key = "Your API key"
import { GoogleGenerativeAI } from "@google/generative-ai";
import { unified } from 'unified';
import remarkParse from 'remark-parse'
import remark2rehype from 'remark-rehype'
import rehypeStringify from 'rehype-stringify';
const genAI = new GoogleGenerativeAI(api_key);
const model = genAI.getGenerativeModel({ model: "gemini-1.5-flash" });
async function generateReply(){
const prompt = document.getElementById("input").value;
const result = await model.generateContent(prompt);
const processor = unified().use(remarkParse).use(remark2rehype).use(rehypeStringify);
const html = processor.processSync(result.response.text());
document.getElementById("output").innerHTML = (html.value);
}
document.getElementById("run").onclick = () => generateReply();
参考にさせていただいたサイト
GitHub - unifiedjs/unified: ☔️ interface for parsing, inspecting, transforming, and serializing content through syntax trees
☔️ interface for parsing, inspecting, transforming, and serializing content through syntax trees - unifiedjs/unified
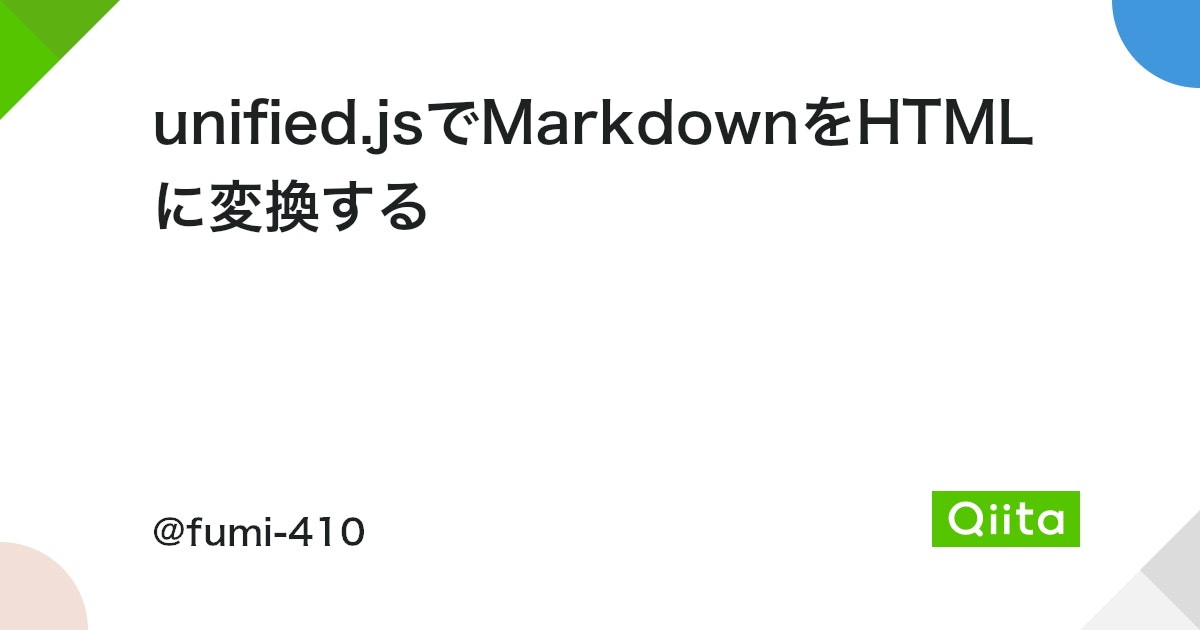
unified.jsでMarkdownをHTMLに変換する - Qiita
unified.jsはAST(抽象構文木)を使いテキストフォーマットを変換できるライブラリです。remark(Markdown)、rehype(HTML)、retext(自然言語)といったフォーマ…
コメント